An Elm REPL for my phone
2024-09-28 (Last updated on 2024-10-31)
I have been building elm.run/repl to write, type-check and compile Elm code on my mobile phone even if I don't have internet access.
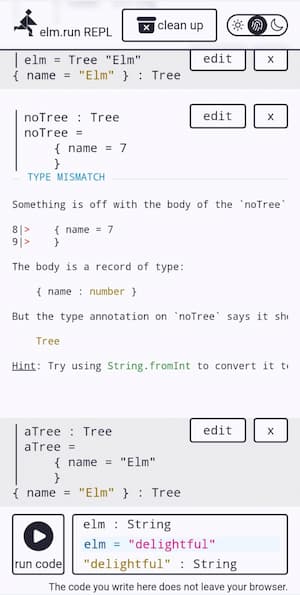
Behavior
As visible in the preview on the left, there is an input area at the bottom where I can enter multiple lines of Elm code. The input field grows as needed, and I tried my best to ensure that it is usually not hidden by the on-screen keyboard on my phone.
With every keystroke, the code is checked and if it is valid I will see the type definition above my input (in the example the declaration elm = "delightful"
) and the evaluated result below, which is the String "delightful".
If I press the "run code" button, it will print the entire compiler output, which is useful for finding errors like in the example where a number
was supplied insted of the expected String
to create a Tree
type alias.
The formatting is supplied by the compiler and looks like it would in the console.
I can scroll up to see older code that I entered, can easily copy each down into the input area with one button press or remove it completely.
It also has a button to execute multiple bulk clean-up operations. From a full reset to removing all outdated declarations to only removing errors, I have multiple options there.
Apart from colors to make the errors and the input field more readable, I tried to keep the amount of distractions low.
It has a light and dark mode, and auto-detects the user's preferences.
For people just starting out, I added an introductory text at the top of the page and a button to insert example code.
Right now it randomly picks one of several examples each with multiple entered expressions.